OpenSCAD code at the bottom of this post.

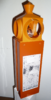


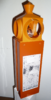

Code:
// Filename: bp1010_lantern.scad
// Author: asicer
// Date: 2022-08-22
phgt=15.0; // plug height
plng=73.0; // plug length
pwdt=60.0; // plug width
nwdt=19.0; // notch width
ndpt= 2.5; // notch depth
lhgt= 2.0; // lid height
shol= 9.0; // screw hole offset from l edge (horiz)
shor=12.7; // screw hole offset from r edge (horiz)
shov= 7.5; // screw hole offset from plug top (vert)
shdi= 3.6; // screw hole diameter
shdp=10.0; // screw hole depth
skdi=18.0; // socket diameter
ohdg=60.0; // maximum overhang (degrees) printer can handle
mfl = 0.01;// manifold (discontinuity)
module plug3() { // outer third of batt plug
difference() {
cube([(pwdt-nwdt)/2,plng,phgt]);
// two screw holes
translate([-mfl,shol,phgt-shov]) rotate([0,90,0]) cylinder($fn=20, h=shdp,d=shdi);
translate([-mfl,plng-shor,phgt-shov]) rotate([0,90,0]) cylinder($fn=20, h=shdp,d=shdi);
}
}
module plug() { // entirety of batt plug
plug3(); // side
translate([pwdt,0,0])mirror([1,0,0]) plug3(); // other side
translate([(pwdt-nwdt)/2,ndpt,0])cube([nwdt,plng-(2*ndpt),phgt]); // middle
translate([0,0,phgt]) cube([pwdt,plng,lhgt]); // lid
}
hsng=pwdt;// housing length/width (square)
htck= 3; // housing wall thickness
lnsw=10; // lens depth
lnsd=hsng-htck; // lens diameter
module lens() {
difference() {
cylinder($fn=90,h=lnsw, d2=lnsd-lnsw, d1=lnsd);
translate([0,0,-mfl])
cylinder(h=lnsw+(3*mfl), d2=lnsd-lnsw-htck, d1=lnsd-htck);
}
}
module tetrahedron(slen){
linear_extrude(height=(slen/2)/tan(ohdg),scale=0)square([slen,slen],center=true);
}
module housing() { // lamp housing
difference() { // bulb surround
cube([hsng,hsng,hsng]);
translate([(hsng/2),hsng+mfl,(lnsd/2)+(htck/2)]) rotate([90,0,0]) cylinder(h=hsng+(2*mfl), d=lnsd-htck);
translate([-mfl,(hsng/2),(lnsd/2)+(htck/2)]) rotate([0,90,0]) cylinder(h=hsng+(2*mfl),d=lnsd-htck);
translate([htck,htck,htck]) cube([hsng-(2*htck),hsng-(2*htck),hsng+mfl]);
}
// lenses
translate([(hsng/2),0,(lnsd/2)+(htck/2)]) rotate([90,0,0]) lens();
translate([hsng,(hsng/2),(lnsd/2)+(htck/2)]) rotate([90,0,90]) lens();
translate([(hsng/2),hsng,(lnsd/2)+(htck/2)]) rotate([90,0,180]) lens();
translate([0,(hsng/2),(lnsd/2)+(htck/2)]) rotate([90,0,-90]) lens();
// roof
translate([hsng/2,hsng/2,hsng])tetrahedron(slen=hsng);
// decorative flourish on top
translate([hsng/2,hsng/2,hsng+((hsng-skdi)/(2*tan(ohdg)))]) cube([skdi,skdi,2*htck], center=true);
translate([(hsng/2),(hsng/2),hsng+htck+((hsng-skdi)/(2*tan(ohdg)))]) cylinder(h=skdi,d1=skdi,d2=skdi*sqrt(3)); // 1:1:sqrt(3) seems like a pleasant ratio
translate([(hsng/2),(hsng/2),hsng+htck+skdi+((hsng-skdi)/(2*tan(ohdg)))]) cylinder(h=skdi/2,d1=skdi*sqrt(3),d2=0); // sqrt(3):0.5 also seems pleasant
}
difference() { // everything put together
union() {
plug();
translate([0,(plng-hsng)/2,phgt+lhgt]) housing();
}
translate([hsng/2,((plng-hsng)/2)+(hsng/2),phgt+lhgt+hsng-mfl])tetrahedron(slen=hsng-(2*htck)); // ceiling
translate([pwdt/2,plng/2,-mfl]) cylinder($fn=40,h=phgt+lhgt+htck+(2*mfl),d=skdi); // socket hole
translate([pwdt/2,plng/2,-mfl]) cylinder($fn=40,h=phgt,d1=skdi+(2*phgt),d2=skdi); // socket relief (45deg conical)
}
Last edited: